WordPress plugins can add powerful features to your website, but sometimes, you may prefer a more lightweight approach, especially when it comes to displaying post view counts. In this guide, we’ll walk through a simple method to showcase your post views without relying on additional plugins.
Step 1
Open Your Theme’s/Childe Theme’s functions.php File.
Access your WordPress theme’s/child theme’s functions.php file. You can find it by navigating to your WordPress dashboard, selecting “Appearance,” then choosing “Theme Editor.” Look for the functions.php file on the right-hand side.
Insert Code Snippet for Post Views.
Add the following code snippet at the end of your functions.php file:
function getPostViews($postID){
$count_key = 'post_views_count';
$count = get_post_meta($postID, $count_key, true);
if($count==''){
delete_post_meta($postID, $count_key);
add_post_meta($postID, $count_key, '0');
return "0 View";
}
return $count.' Views';
}
function setPostViews($postID) {
$count_key = 'post_views_count';
$count = get_post_meta($postID, $count_key, true);
if($count==''){
$count = 0;
delete_post_meta($postID, $count_key);
add_post_meta($postID, $count_key, '0');
}else{
$count++;
update_post_meta($postID, $count_key, $count);
}
}
This code establishes two functions – getPostViews to retrieve post views and setPostViews to update them.
Step 2
Display Post Views in Your Theme
Now, wherever you want to display the post views on your site, insert the following code:
<?php echo getPostViews(get_the_ID()); ?>
You can add this code within your theme files, such as single.php or content.php, where you want the post views to appear.
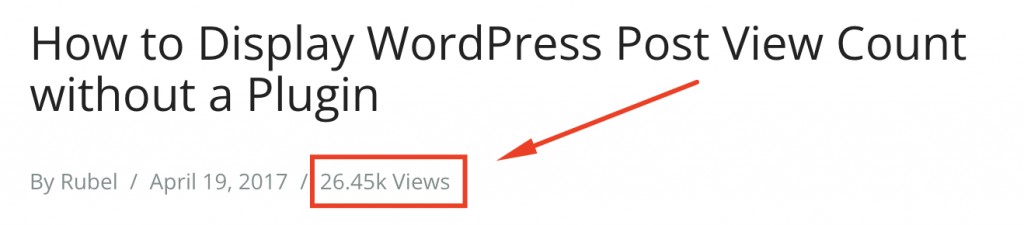
By following these steps, you’ve successfully implemented a basic post view count feature without relying on a plugin, providing a lightweight and customizable solution for your WordPress site.
Leave a Reply